Debugging Using Conditional Breakpoints & fprintf
Latest activity Reply by Duncan Carlsmith
on 22 Apr 2024
Temporary print statements are often helpful during debugging but it's easy to forget to remove the statements or sometimes you may not have writing privileges for the file. This tip uses conditional breakpoints to add print statements without ever editing the file!
What are conditional breakpoints?
Conditional breakpoints allow you to write a conditional statement that is executed when the selected line is hit and if the condition returns true, MATLAB pauses at that line. Otherwise, it continues.
The Hack: use ~fprintf() as the condition
fprintf prints information to the command window and returns the size of the message in bytes. The message size will always be greater than 0 which will always evaluate as true when converted to logical. Therefore, by negating an fprintf statement within a conditional breakpoint, the fprintf command will execute, print to the command window, and evalute as false which means the execution will continue uninterupted!
How to set a conditional break point
1. Right click the line number where you want the condition to be evaluated and select "Set Conditional Breakpoint"
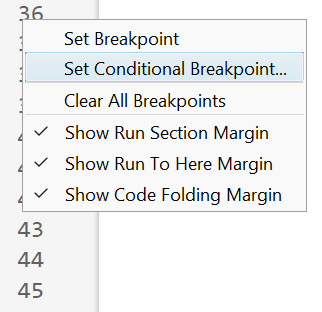
2. Enter a valid MATLAB expression that returns a logical scalar value in the editor dialog.

Handy one-liners
Check if a line is reached: Don't forget the negation (~) and the line break (\n)!
~fprintf('Entered callback function\n')
Display the call stack from the break point line: one of my favorites!
~fprintf('%s\n',formattedDisplayText(struct2table(dbstack)))
Inspect variable values: For scalar values,
~fprintf('v = %.5f\n', v)
~fprintf('%s\n', formattedDisplayText(v)).
Make sense of frequent hits: In some situations such as responses to listeners or interactive callbacks, a line can be executed 100s of times per second. Incorporate a timestamp to differentiate messages during rapid execution.
~fprintf('WindowButtonDownFcn - %s\n', datetime('now'))
Closing
This tip not only keeps your code clean but also offers a dynamic way to monitor code execution and variable states without permanent modifications. Interested in digging deeper? @Steve Eddins takes this tip to the next level with his Code Trace for MATLAB tool available on the File Exchange (read more).
Summary animation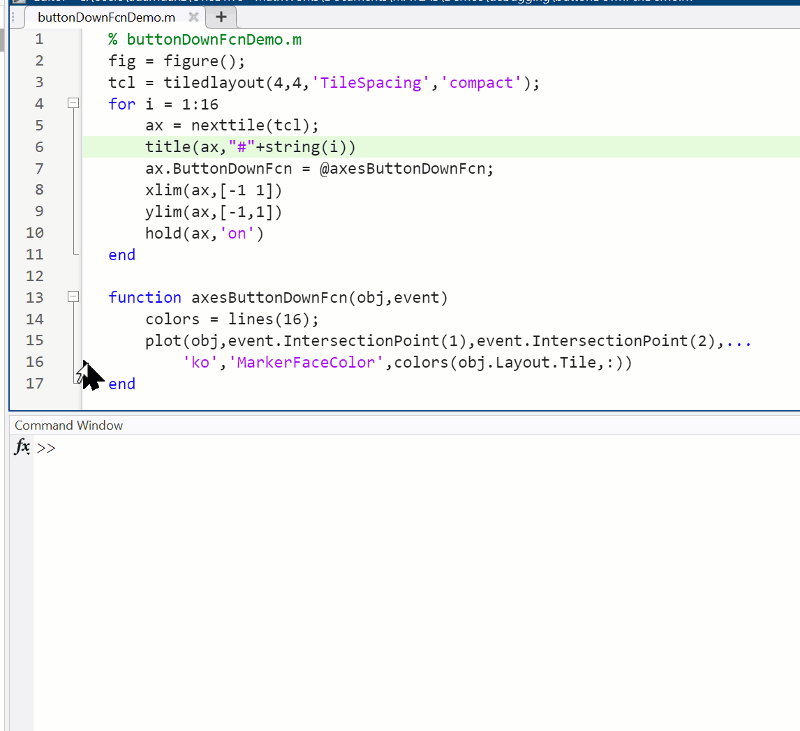
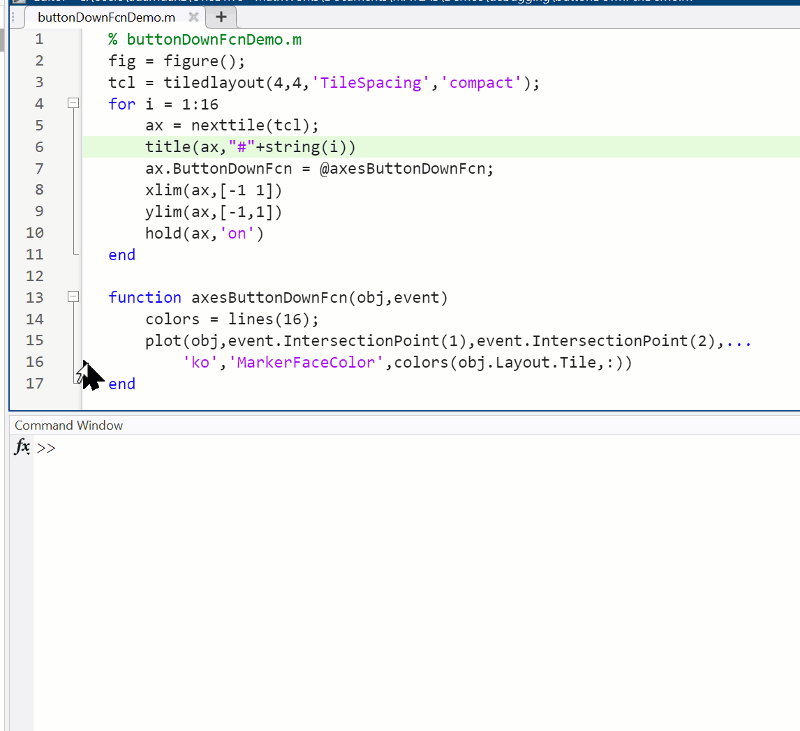
To reproduce the events in this animation:
% buttonDownFcnDemo.m
fig = figure();
tcl = tiledlayout(4,4,'TileSpacing','compact');
for i = 1:16
ax = nexttile(tcl);
title(ax,"#"+string(i))
ax.ButtonDownFcn = @axesButtonDownFcn;
xlim(ax,[-1 1])
ylim(ax,[-1,1])
hold(ax,'on')
end
function axesButtonDownFcn(obj,event)
colors = lines(16);
plot(obj,event.IntersectionPoint(1),event.IntersectionPoint(2),...
'ko','MarkerFaceColor',colors(obj.Layout.Tile,:))
end
2 Comments
Nice.
That is a slick trick! Especially since breakpoints can be disabled (i.e., when not debugging) but still retained in the file for later use.
Also, a trailing line break is not needed if strings are used instead of character arrays.